As Large Language Models (LLMs) continue to revolutionize the field of natural language processing, organizations are increasingly looking for ways to adapt these powerful tools to their specific needs. Enter LLM fine-tuning – a technique that allows us to customize pre-trained models for specialized tasks and domains. In this blog post, we'll explore the world of LLM fine-tuning, its value, and how you can implement it in your own projects.
Summary
- LLM fine-tuning is the process of adapting pre-trained language models to specific tasks or domains.
- Fine-tuning offers improved performance, reduced computational costs, and customization for niche applications.
- Common use cases include sentiment analysis, named entity recognition, and domain-specific text generation.
- Implementing fine-tuning is accessible through libraries like Hugging Face's Transformers.
- Ethical considerations and best practices are crucial when fine-tuning LLMs.
What is LLM Fine-Tuning?
LLM fine-tuning is a transfer learning technique that involves taking a pre-trained language model and further training it on a smaller, task-specific dataset. This process allows the model to adapt its knowledge to a particular domain or task while retaining the general language understanding it acquired during pre-training.
Fine-tuning typically involves:
- Selecting a pre-trained model (e.g., BERT, GPT-3, T5)
- Preparing a task-specific dataset
- Adjusting the model's parameters on the new data
- Evaluating and iterating on the fine-tuned model
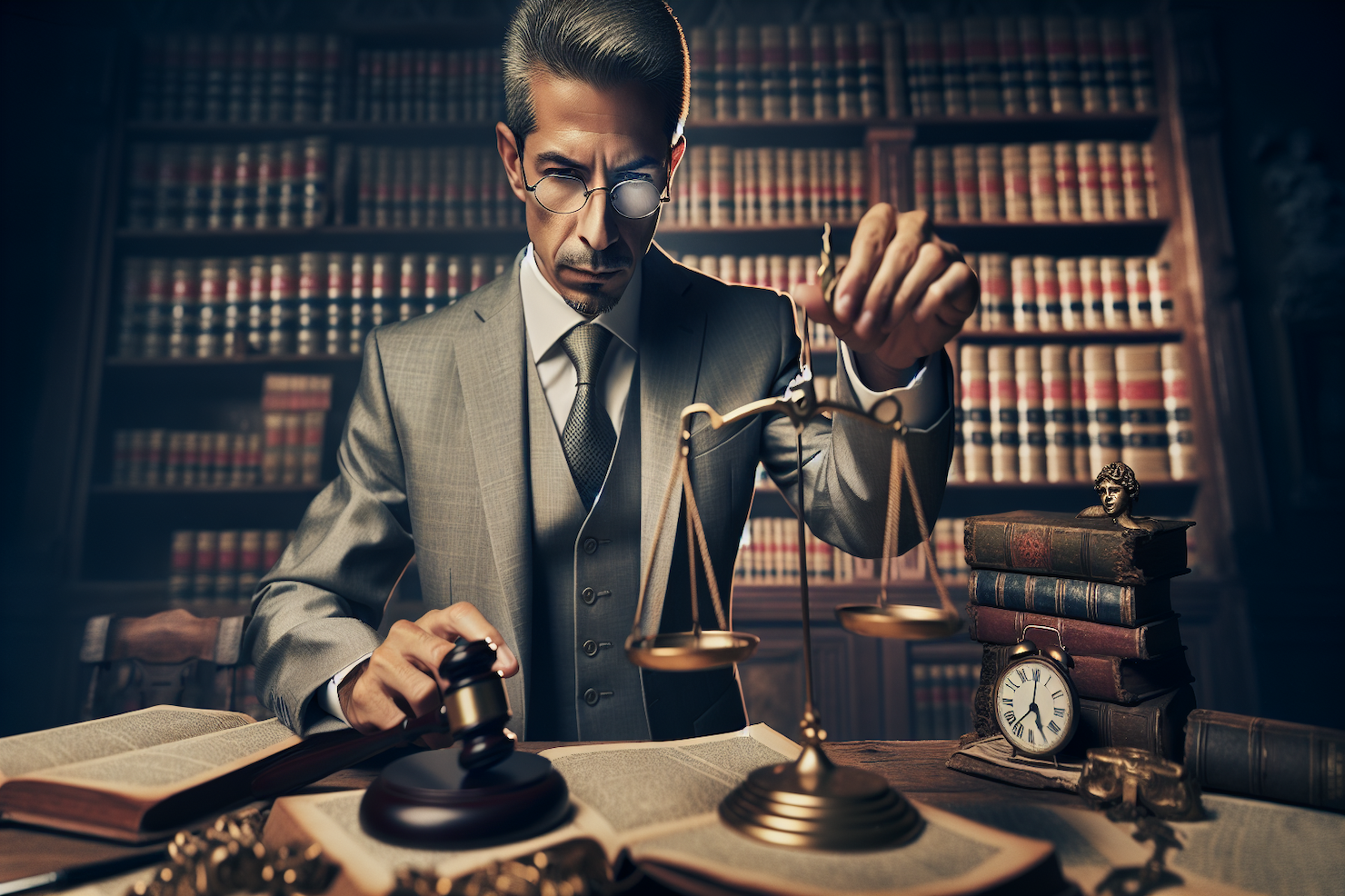
The Value of Fine-Tuning
Fine-tuning LLMs offers several key advantages:
- Improved Performance: Fine-tuned models often outperform general-purpose models on specific tasks.
- Resource Efficiency: Fine-tuning requires less data and computational resources than training from scratch.
- Customization: Allows adaptation to niche domains or specialized vocabularies.
- Rapid Prototyping: Enables quick development of task-specific models for various applications.
- Continuous Learning: Facilitates updating models with new information or adapting to shifting data distributions.
Example Use Cases
Fine-tuning LLMs has proven valuable across numerous applications:
- Sentiment Analysis: Tailoring models to understand industry-specific sentiment in customer reviews or social media.
- Named Entity Recognition: Adapting models to recognize domain-specific entities in legal, medical, or financial texts.
- Text Classification: Improving categorization of documents for content moderation or topic sorting.
- Question Answering: Enhancing models to provide accurate answers in specific knowledge domains.
- Language Translation: Refining translation models for niche languages or technical vocabularies.
- Text Generation: Customizing models to produce domain-specific content, such as product descriptions or technical documentation.
Implementing Fine-Tuning with Hugging Face and Python
Let's walk through a simple example of fine-tuning a BERT model for sentiment analysis using the Hugging Face Transformers library:
import torch
from transformers import BertTokenizer, BertForSequenceClassification, Trainer, TrainingArguments
from datasets import load_dataset
# Load pre-trained model and tokenizer
model_name = "bert-base-uncased"
tokenizer = BertTokenizer.from_pretrained(model_name)
model = BertForSequenceClassification.from_pretrained(model_name, num_labels=2)
# Load and preprocess the dataset
dataset = load_dataset("imdb")
def tokenize_function(examples):
return tokenizer(examples["text"], padding="max_length", truncation=True)
tokenized_datasets = dataset.map(tokenize_function, batched=True)
# Define training arguments
training_args = TrainingArguments(
output_dir="./results",
num_train_epochs=3,
per_device_train_batch_size=8,
per_device_eval_batch_size=8,
warmup_steps=500,
weight_decay=0.01,
logging_dir="./logs",
)
# Initialize Trainer
trainer = Trainer(
model=model,
args=training_args,
train_dataset=tokenized_datasets["train"],
eval_dataset=tokenized_datasets["test"],
)
# Fine-tune the model
trainer.train()
# Save the fine-tuned model
model.save_pretrained("./fine_tuned_bert_sentiment")
tokenizer.save_pretrained("./fine_tuned_bert_sentiment")
This script demonstrates the basic steps of fine-tuning a BERT model on the IMDB dataset for sentiment analysis. It leverages Hugging Face's Transformers library to simplify the process of loading pre-trained models, preparing data, and training.
Best Practices and Considerations
When fine-tuning LLMs, keep these best practices in mind:
- Data Quality: Ensure your fine-tuning dataset is high-quality, diverse, and representative of the target task.
- Hyperparameter Tuning: Experiment with learning rates, batch sizes, and other hyperparameters to optimize performance.
- Evaluation: Regularly evaluate your model on a held-out test set to prevent overfitting.
- Ethical Considerations: Be aware of potential biases in your training data and take steps to mitigate them.
- Resource Management: Balance the trade-off between model size, performance, and computational requirements.
Conclusion
Fine-tuning Large Language Models represents a powerful technique for adapting state-of-the-art NLP models to specific tasks and domains. By leveraging pre-trained models and fine-tuning them on specialized datasets, organizations can achieve high-performance, custom language models with relatively modest resources.
At A42 Labs, we're excited about the potential of fine-tuned LLMs to solve complex language tasks across various industries. Our team of experts is dedicated to helping organizations implement these cutting-edge techniques to drive innovation and efficiency in their natural language processing pipelines.
As the field of NLP continues to evolve, fine-tuning will play an increasingly important role in bridging the gap between general-purpose language models and specialized applications. By mastering this technique, data scientists and machine learning engineers can unlock new possibilities in text analysis, generation, and understanding.
If you're interested in learning more about how A42 Labs can help your organization leverage LLM fine-tuning for your specific needs, please reach out to us at info@a42labs.io.
References
- Howard, J., & Ruder, S. (2018). Universal Language Model Fine-tuning for Text Classification. arXiv preprint arXiv:1801.06146. https://arxiv.org/abs/1801.06146